Essential Software Development Tools for Modern Developers
Software development is a complex and evolving field that requires developers to use a variety of tools to create, debug, test, and maintain applications. Whether you’re a seasoned software engineer or just starting in the world of coding, the right software development tools can significantly enhance your productivity, code quality, and workflow.
In this article, we’ll explore essential software development tools that every developer should know about. From integrated development environments (IDEs) to version control systems and debugging tools, these tools help streamline the development process and ensure that your projects are built with efficiency and quality in mind.
1. Integrated Development Environments (IDEs)
IDEs are one of the most important tools for software developers. They provide a comprehensive environment to write, debug, and test code. IDEs typically come with features like code completion, syntax highlighting, debugging tools, and built-in compilers. Here are a few popular ones:
1.1. Visual Studio Code (VSCode)
VSCode is a lightweight yet powerful source code editor developed by Microsoft. It’s open-source and highly customizable, with a large extension marketplace for adding new features and languages.
Key Features:
- Multi-language Support: Supports a variety of languages such as JavaScript, Python, C++, and more.
- Extensions: Customize your editor with extensions for linting, code formatting, version control, and more.
- Integrated Terminal: Includes a terminal for running commands directly within the IDE.
Why It’s Great:
- Free and Open-Source: Available for free on all major platforms (Windows, macOS, Linux).
- Highly Customizable: You can install extensions to tailor the editor to your specific workflow.
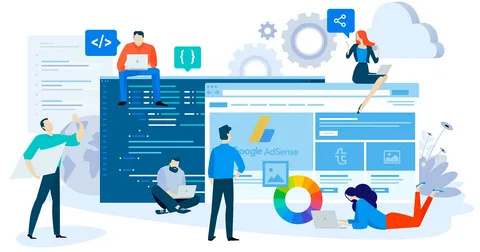
1.2. IntelliJ IDEA
IntelliJ IDEA is a robust IDE for Java developers, although it supports multiple languages, including Kotlin, Groovy, and Scala. Known for its intelligent code completion and refactoring tools, IntelliJ is favored by developers who need a highly efficient, Java-centric development environment.
Key Features:
- Advanced Code Assistance: Features like real-time code analysis, code suggestions, and quick fixes.
- Integrated Tools: Built-in tools for version control, databases, and deployment.
- Refactoring Support: Helps developers refactor code without breaking the project.
Why It’s Great:
- Smart Code Assistance: IntelliJ’s ability to suggest optimizations and identify potential issues in your code is exceptional.
- Comprehensive Features: It integrates seamlessly with other tools like Docker and Kubernetes.
1.3. Eclipse
Eclipse is one of the most popular IDEs for Java development. It is an open-source, extensible platform that offers tools for Java, C/C++, PHP, and more. It’s often used for building complex, large-scale applications.
Key Features:
- Modular: Eclipse is highly extensible, allowing developers to add plugins for various languages and tools.
- Version Control: Supports Git, Subversion, and CVS for version control.
- Rich Plugin Ecosystem: A wide variety of plugins are available to integrate with databases, testing frameworks, and more.
Why It’s Great:
- Open-Source: Free to use with a large community of contributors.
- Enterprise-Ready: Ideal for building enterprise-level applications and handling large codebases.
2. Version Control Systems (VCS)
Version control systems (VCS) are essential for tracking changes in your codebase and collaborating with other developers. They allow you to revert to previous versions, manage branches, and resolve conflicts that arise from multiple contributors.
2.1. Git
Git is the most widely used version control system today. It is a distributed version control system, meaning each developer has a local copy of the entire repository. This allows for fast operations and better branching strategies.
Key Features:
- Branching and Merging: Easily create branches to experiment with new features or fixes without affecting the main codebase.
- Distributed: Each developer works on their own copy of the code, improving performance and collaboration.
- GitHub and GitLab Integration: Git is integrated with platforms like GitHub and GitLab, which offer remote repositories and collaborative tools.
Why It’s Great:
- Widely Adopted: Git is the industry standard and is used by developers across the world.
- Strong Community: With widespread use, Git offers a vast amount of resources, tutorials, and documentation.
2.2. GitHub
While Git is the underlying version control system, GitHub is a platform that hosts Git repositories and provides additional collaboration features. GitHub is essential for managing open-source projects, sharing code with others, and working in teams.
Key Features:
- Pull Requests: Developers can submit pull requests to propose changes, which can be reviewed and merged by others.
- Issue Tracking: GitHub allows you to track bugs, features, and tasks, making project management easier.
- Actions: Automate your workflow with GitHub Actions for continuous integration and deployment (CI/CD).
Why It’s Great:
- Collaboration: GitHub’s collaborative features make it perfect for teams and open-source contributions.
- Free Tier: GitHub offers a generous free plan, making it accessible for individuals and small teams.
3. Testing Tools
Testing is an integral part of software development. Automated testing frameworks and tools ensure your code works as expected and prevent bugs from being introduced into the system.
3.1. Selenium
Selenium is a powerful tool for automating web browsers. It’s primarily used for writing functional tests for web applications, simulating user interactions with the site.
Key Features:
- Cross-Browser Testing: Selenium supports all major browsers, including Chrome, Firefox, and Internet Explorer.
- Language Support: Works with programming languages like Java, Python, C#, and JavaScript.
- WebDriver: The Selenium WebDriver allows interaction with web elements directly, making it ideal for functional testing.
Why It’s Great:
- Open-Source: Selenium is free to use, with a strong community of developers.
- Cross-Platform: Selenium tests can run on different operating systems and browsers.
3.2. JUnit
JUnit is a widely used framework for writing unit tests in Java. It provides annotations to define test cases and assertions to verify the expected outcomes.
Key Features:
- Simple Syntax: JUnit’s syntax is simple and easy to learn, making it accessible for both beginners and experienced developers.
- Integration with IDEs: Most popular Java IDEs like Eclipse and IntelliJ IDEA have built-in support for JUnit.
- JUnit 5: The latest version of JUnit provides advanced features like parameterized tests and extensions.
Why It’s Great:
- Standard for Java Testing: JUnit is the de facto standard for unit testing in Java applications.
- Automation: JUnit tests can be integrated into CI/CD pipelines for automated testing.
4. Database Management Tools
Managing databases is a crucial aspect of many software development projects. From designing the schema to querying data and optimizing performance, database management tools help streamline this process.
4.1. MySQL Workbench
MySQL Workbench is a unified visual tool that provides database design, SQL development, and database administration for MySQL databases.
Key Features:
- SQL Development: Write and execute SQL queries directly in the editor.
- Database Design: Use the visual tools to design database schemas and create ER diagrams.
- Data Migration: MySQL Workbench offers features to migrate data from other database systems to MySQL.
Why It’s Great:
- Integrated Toolset: Combines multiple functions into one tool, making it ideal for MySQL developers.
- Free: MySQL Workbench is free and open-source, with a comprehensive set of features.
4.2. MongoDB Compass
MongoDB Compass is the official graphical user interface (GUI) for MongoDB, one of the most popular NoSQL databases. It provides an easy way to query, visualize, and manage data in MongoDB.
Key Features:
- Schema Visualization: Visualize your data schema and understand how your collections are structured.
- Query Builder: Use a simple GUI to build and execute MongoDB queries.
- Aggregation Pipeline: Work with the powerful MongoDB aggregation pipeline for advanced queries.
Why It’s Great:
- User-Friendly: MongoDB Compass simplifies working with MongoDB, especially for users who prefer a graphical interface.
- Real-Time Insights: Helps you analyze and troubleshoot your MongoDB data more efficiently.
5. DevOps and CI/CD Tools
DevOps tools automate and streamline the software development lifecycle, particularly continuous integration (CI) and continuous deployment (CD). These tools help developers deploy code faster and with fewer errors.
5.1. Jenkins
Jenkins is an open-source automation server commonly used for continuous integration and continuous deployment (CI/CD). It automates the process of building, testing, and deploying code.
Key Features:
- Pipeline Automation: Jenkins allows you to automate complex workflows, from testing to deployment.
- Plugins: A vast collection of plugins enables Jenkins to integrate with other tools like Git, Maven, Docker, and Kubernetes.
- Distributed Builds: Jenkins supports distributed builds, improving scalability and speed.
Why It’s Great:
- Flexible: Jenkins can be tailored to suit almost any project or team with its extensive plugin ecosystem.
- Free and Open-Source: Jenkins is completely free to use, with a large community of developers contributing to its growth.
Conclusion
The tools a developer uses can significantly impact productivity, code quality, and collaboration. From IDEs to version control systems, testing frameworks, and DevOps tools, the right combination of software development tools enables developers to work more efficiently, create better products, and reduce errors.
By incorporating the tools mentioned above into your workflow, you’ll be equipped to handle the complexities of modern software development while maintaining a high level of quality and productivity. Whether you’re building web applications, mobile apps, or enterprise-level software, these tools can provide the support you need to succeed.